Message passing between tasks
The SDR Virtual Machine supports multitasking and each script running has its own private memory where the variables are stored. In many situations it is required to exchange data between tasks.
For example one task could be monitoring the activities over the received band while another one is running a narrowband receiver and needs instructions to retune its Digital Downconverter.
The SDR Virtual Machine comes with two different ways to pass data from one task to another :
- A Mailbox API,
- A Shared Map API.
Introduction to Mailboxes
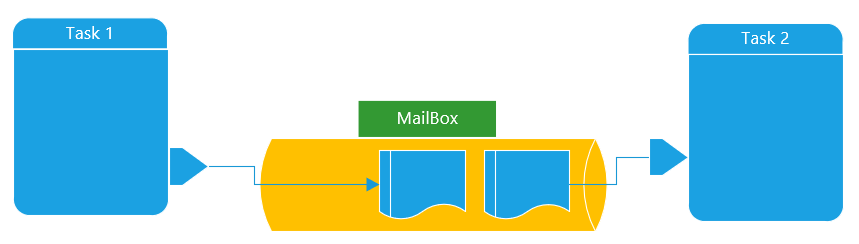
The name of the Mailbox is unique and each task can get a reference to the Mailbox by using its name.
bool result = MBoxCreate(mailbox_name) ;
This call will return true is the mailbox has been created, false it was already existing. Now that we are sure the mailbox exists, we can post or read messages (JSON objects), test if messages are available etc. For more details read the full API here.
The following code snippet shows different examples :
// Create MBoxCreate('box'); // Post an number MBoxPost('box', 1 ); // Post a string MBoxPost('box', 'test string' ); // Post an object MBoxPost('box', {'x': 3} );
The following example shows a simple “ping-pong” task, waiting for the “ping” and sending back a pong :
print('Pong task starting'); for( var i=0 ; i < 100 ; i++ ) { var msg = MBoxPopWait('ping', 10000); // We wait up to 10 seconds print('Pong:: received message : ' + JSON.stringify( msg ) ); MBoxPost('pong','ok'); } print('Task pong ends');
Connecting a Mailbox to MQTT
The SDR Virtual Machine embeds a MQTT client that can be directly connected to the MailBox at creation time by passing an additional JSON object defining the MQTT server address and login parameters. Any message arriving on the selected topic will be available in the mailbox.
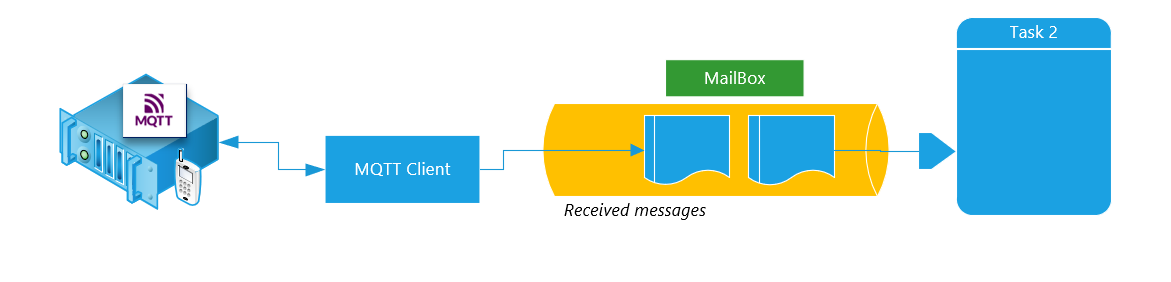
The following code shows a simple example :
// Create a box that is connected to MQTT var config = { 'host': 'localhost', 'login': 'mqtt', 'pass' : 'mqtt', 'topic': 'test' } ; if( MBoxCreate('box', config) == false ) { if( MBoxExists('box') == false ) { print('could not connect'); exit(); } } var content = '123456789' ; MBoxPost('box',content ) ;
Now that the mailbox is connected to MQTT :
- Messages send in the mailbox will be forwarded to the MQTT server on the given topic,
- Messages received on the given topic will be pushed to the mailbox incoming queue and can be read using the standard API.
Comments are closed